- March 8, 2023
- Posted by: Maksim Zavarin
- Category: Blog
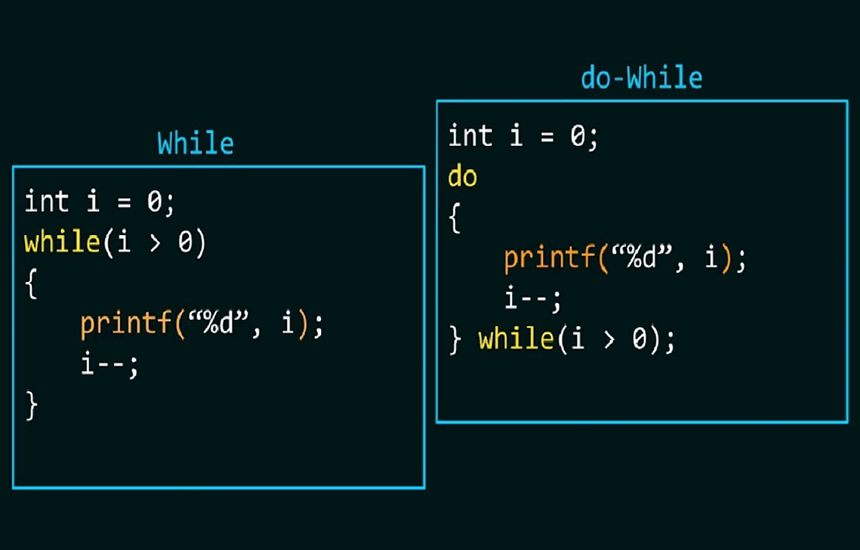
What Is the Difference Between While and Do-While Loop in C?
This article contains everything you need to know about while and do-while loops, including the syntax, flow charts, and their key differences.
Loops make programming so much easier since you can repeatedly execute a function until certain conditions are met. If you’re a C programmer, the two most common loops you’re likely to use are while loops and do-while loops. Each has its place in your projects, and naturally, there are several differences between them. If you want to learn more about the difference between a while and do-while loop in C, keep reading. As a side note, both loops are also prominent in Java and C++ programming, so if you’re learning any of these languages, they should come in handy.
What Are Loops?
A loop is typically a sequence of instructions or a “control structure” used to repeatedly execute a section of code until a stopping condition is met. That way, instead of entering the sequence of instructions 1000 times to complete a task, you could write one section of code and use it repeatedly. The stopping condition, typically included in the Boolean condition part of the loop structure, comes in handy to ensure it doesn’t go through infinite iterations.
The loop structure is divided into two parts, i.e., the body and the control statement/Boolean condition. The body consists of the instructions, while the control statement includes the conditions for the body that typically evaluate as either true or false. If it evaluates as true, the code can be executed another time. If it evaluates as false, it typically means the loop is terminated.
Apart from while and do while, loops can also be divided into entry-controlled and exit-controlled loops. With the former, the control statement is included before the body, while with exit controlled loops, the control statement comes after the body of the loop.
Now to learn more about loops and coding in general, you might need to pick up a book or two on the subject. We’ve already found some of the best programming books available for different skill levels, whether newbies, intermediates, or those that cater to more experienced programmers.
However, these recommendations are more generalized, so if you’re looking to learn more about a specific language, e.g., Java, the best Java books may be better suited to your needs.
While loop
A while loop in C programming is an excellent example of an entry-controlled loop with the condition typically placed at the beginning of the loop’s structure. With that, the condition is checked before the execution of the body. After that, the loop body should be executed as often as the condition remains true and will stop as soon as it doesn’t.
Since the condition is checked before each iteration, and the loop can be terminated before the statement execution if it’s not met. Middle Tennessee State University also notes that there are different types of while loops, e.g., flag-controlled while loops, nested while loops, end-of-file-controlled while loops, etc.
Do-while loop
As for the do-while loop, it’s an exit-controlled loop meaning the condition is placed after the body of the loop. As such, the code execution comes first before the condition is checked. However, just like the while loop, the loop body will be executed repeatedly until the condition becomes false. At this point, the loop will be terminated.
Since do-while loops execute the code in the body before checking the condition, you’ll get at least one iteration of code execution even if the condition is false. With while loops, the condition being checked before the body of the loop means that the loop can be terminated even before a single iteration.
If you’d like to learn more about C programming and the different loop types, the C Programming Language, 2nd Edition, is available both as a paperback and a Kindle e-book.
Also, below we include an outline of the syntax of while and do-while loops.
Syntax
While loop in C
while(Boolean condition)
{
Loop statements/body
}
As you can see, the Boolean condition precedes the loop statements/the body.
Do-while loop in C
do
{
Loop statements/body
} while(Boolean condition);
We will get into some differences in the actual loop statements later in the article.
Flow Charts and Explanation
While loop
In the case of the while loop, it starts with condition checking, and if true, the loop statement/body is executed before going back to condition checking. Naturally, if the condition is false the first time the condition is checked, the process is terminated and never gets to statement execution. Here’s a flowchart to better explain the process.
Do-while loop
With do-while loops, you get loop statement execution first, followed by condition checking. If the condition is true, the loop statement will continue to be executed repeatedly until, eventually, it’s false. At this point, instead of the statement being executed again, the loop will be terminated, and here’s a flow chart to show how that goes.
While vs. Do-While Loop: Key Differences
While |
Do-While |
|
|
|
|
|
|
|
|
|
|
FAQ
What is the difference between while and do-while in Java?
In Java, the difference between while and do-while loops is the same as in C programming. A while loop will execute a statement depending on whether the loop condition allows it. Consequently, there could be as few as zero iterations.
With a do-while loop, the fact that the condition is checked after the first iteration or the first statement execution means there are going to be one or more iterations.
What is a for loop?
According to the University of Utah, for loops are another type of entry-controlled loop where the code execution will be repeated a predetermined number of times. In the case of while and do while loops, the number of iterations is undetermined. As such, if the condition checking never evaluates to false, it could theoretically keep executing the statement an infinite number of times.
Final Thoughts
Loops are a relatively basic aspect of programming in C, C++, and Java; having them in your arsenal is essential. However, you’d need to know how to tell them apart so you know when to use one or the other for the intended functionality. Among the most easily confused loops are while and do while loops. So, what’s the difference between while and do while loops? The article above explains the differences sufficiently, even including flowcharts to make them easier to understand.
However, to summarize, while loops are entfry-controlled while do-while loops are exit controlled. Also, in the former, the condition is checked before statement execution, while in the latter, condition checking follows statement execution. Naturally, there are structural differences between the two types of loops as well.
References
- https://www.cs.mtsu.edu/~xyang/2170/whileloops.html
- https://users.cs.utah.edu/~germain/PPS/Topics/for_loops.html